插入排序(Insertion Sort)是一种简单直观的排序算法。它的工作原理是通过构建有序序列,对于未排序数据,在已排序序列中从后向前扫描,找到相应位置并插入。
插入排序(Insertion Sort)
基本原理
每步将一个待排序的元素,将其插入前面已排好序的部分中,直到全部插入完为止。
算法步骤
- 将待排序序列第一个元素看做已排序序列,把第二个元素到最后一个元素当成是未排序序列
- 从未排序序列中取出下一个元素记为 a,在已排序的序列中从后向前扫描
- 如果该元素(已排序)大于 a,将该元素移到下一位置
- 重复步骤 3,直到找到已排序的元素小于或者等于 a 的位置
- 将 a 插入到该位置后
- 重复步骤 2~5,直至没有未排序元素
动画演示
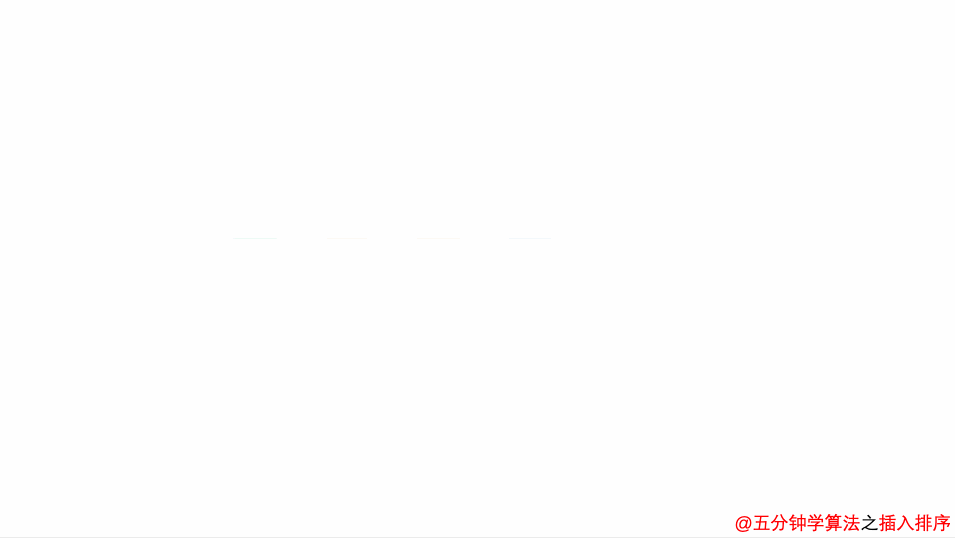
参考实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| import java.util.Arrays;
public class InsertSort { public static void sort(int[] arr) { for (int i = 1; i < arr.length; i++) { int j = i, tmp = arr[i]; for (; j >= 1 && arr[j - 1] > tmp; j--) { arr[j] = arr[j - 1]; } arr[j] = tmp; } }
public static void main(String[] args) { int[] arr = {3, 1, 4, 9, 6, 0, 7, 2, 5, 8}; InsertSort.sort(arr); System.out.println(Arrays.toString(arr)); } }
|
复杂度分析
插入排序 |
\(O(n^2)\) |
\(O(n)\) |
\(O(n^2)\) |
\(O(1)\) |
In-place |
稳定 |
References
https://en.wikipedia.org/wiki/Insertion_sort
https://youliao.163yun.com/api-server/rss/xiaomi/item/IN2WDOMKAWVNTZV.html?ref=browser_news&s=mb&cp=cn-netease-youliao-browser&docid=44797c69e120935b2c4790d933d02a9b&itemtype=news&cateCode=rec&category=%E7%A7%91%E6%8A%80